Streamline Complex Imports in Odoo
Odoo’s built-in import tools are great for quick data loads, but they don’t always fit custom workflows, especially when dealing with complex models or when you need to update existing records in bulk based on custom logic.
In this tutorial, We'll build a custom import wizard in Odoo that lets users to upload an Excel (.xlsx) file to automatically update fields like Serial Number, IMEI 1, and IMEI 2 for each line item in a custom Bulk Purchase model, matched via a unique field like ISN (Item Serial Number).
Why a Custom Import Wizard?
Imagine you have a custom model named purchase.bulk.item that holds detailed product entries in a purchase order. After receiving your products, the supplier provides an Excel file containing:
- ISN (Item Serial Number)
- Serial Number
- IMEI 1
- IMEI 2
Manually entering these details for dozens or hundreds of items in the Odoo interface would be time-consuming and error-prone. So, we’ll create a user-friendly import wizard that automates this process, updating records accurately with just a file upload.
Step-by-Step Implementation
1. Create the Wizard Model
- This model is a TransientModel — used for temporary data like wizards.
- It accepts a binary file field, decodes it, reads rows, and updates matching records in the database based on the ISN.
2. Create the Wizard View
The wizard shows a simple form with a file upload field and two buttons — Import and Cancel.
3. Add an Action & Menu
You can attach this wizard to a smart button in the purchase order or product form, depending on your workflow.
4. Add Button Access
Example of adding a button on the purchase.bulk.item form view:
Testing the Wizard
To verify that everything works:
- Create a few purchase.bulk.item records with known ISN values.
- Prepare an Excel file with headers: ISN, Serial No, IMEI 1, IMEI 2.
- Go to the wizard and upload your file.
- Click Import and confirm that the matching records are updated in Odoo.
Wizard View

Sample Excel Format
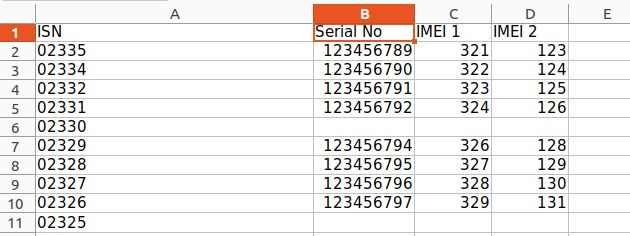
Wrap-Up
Custom import wizards in Odoo are a powerful way to automate data processing, especially when the default tools just don’t cut it.
With a bit of Python and XML, you can create user-friendly tools that:
- Speed up operations
- Reduce human error
- Improve data integrity
- Impress clients with professional polish
This technique is widely applicable across domains: sales, HR, inventory, education, and even finance.
Found this article useful?
Explore more development guides and solutions from our team.